Understanding Timestamp to Date Conversion using JavaScript
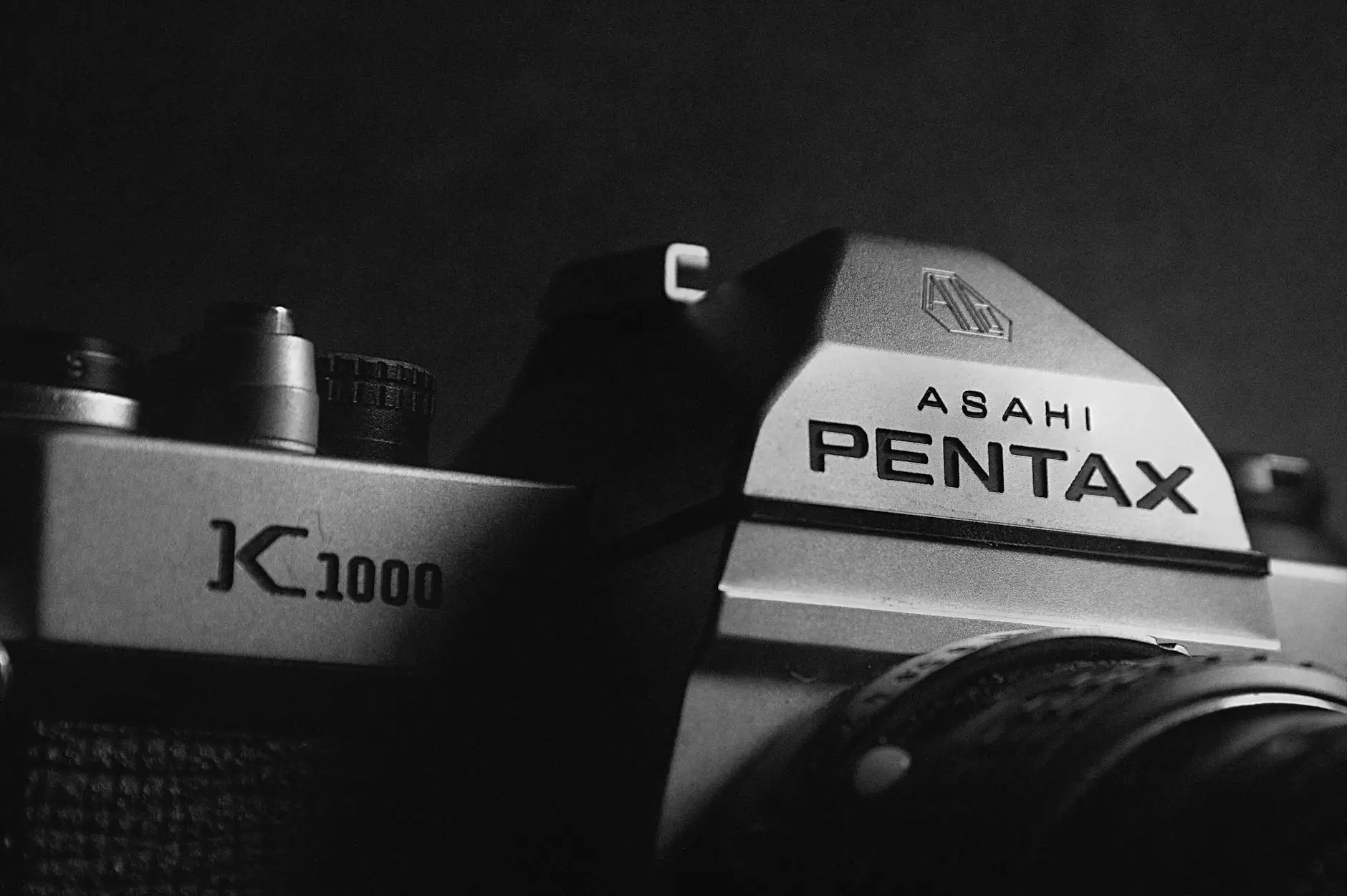
The advent of technology has revolutionized how businesses operate, especially in the fields of Web Design and Software Development. One common task that developers encounter is converting a timestamp to a human-readable date format. This article provides a deep dive into how you can master this essential skill using JavaScript.
What is a Timestamp?
A timestamp is a sequence of characters or encoded information that represents a specific date and time. It is often expressed in numeric format, measuring the time elapsed since a reference point, known as the Unix Epoch, which is set at January 1, 1970, at 00:00:00 UTC.
The Importance of Timestamps in Development
- Data Storage: Timestamps are crucial for recording the exact time an event occurs.
- Logging: They aid developers in tracking changes and debugging systems.
- Time Zones: Timestamps help in maintaining consistency across different geographical locations.
How Timestamps are Represented in JavaScript
In JavaScript, timestamps are typically expressed as the number of milliseconds since the Unix Epoch. This numeric representation provides a compact means of storing time and is a common format used in Web Design and Software Development.
Converting Timestamp to Date in JavaScript
The most straightforward way to convert a timestamp to a date in JavaScript is by using the Date object. The following is a methodical process that entails creating a Date object and formatting it as needed:
let timestamp = 1633072800000; // Example timestamp let date = new Date(timestamp); console.log(date.toString()); // Outputs: 'Mon Oct 01 2021 00:00:00 GMT+0000 (Coordinated Universal Time)'Common Methods for Date Formatting
JavaScript provides various methods for manipulating dates. Here are some of the most commonly used methods:
- toString() - Converts the date to a string representation in the local time zone.
- toISOString() - Converts the date to a string using the ISO format (YYYY-MM-DDTHH:mm:ss.sssZ).
- toUTCString() - Converts the date to a string in UTC format.
- getFullYear(), getMonth(), getDate() - Get specific components of the date.
Examples of Converting Timestamps to Dates
Example 1: Basic Conversion
The following code snippet demonstrates a basic conversion from a timestamp to a date string:
let timestamp = Date.now(); // Get current timestamp let date = new Date(timestamp); console.log("Current Date and Time: " + date.toString());Example 2: Formatting a Date
In this example, we format the date to a more readable format:
let timestamp = 1633072800000; let date = new Date(timestamp); let options = { year: 'numeric', month: 'long', day: 'numeric' }; console.log(date.toLocaleDateString('en-US', options)); // Outputs: 'October 1, 2021'Handling Time Zones
When working with timestamps and dates, it is vital to consider the impact of time zones. JavaScript's Date object defaults to the local time zone when creating dates from timestamps. To manage this effectively, you can convert UTC timestamps directly:
let timestamp = 1633072800000; let dateUTC = new Date(timestamp); console.log("Current Date in UTC: " + dateUTC.toUTCString());Best Practices for Date Handling in JavaScript
To ensure robust and maintainable code when handling timestamps and dates, consider the following best practices:
- Always work in UTC: This prevents confusion and discrepancies when handling dates across different time zones.
- Use libraries: For more complex date manipulation, consider using libraries such as Moment.js or the native DateTime API.
- Validation: Always validate your timestamps before converting to avoid potential runtime errors.
Integrating Date Conversion in Your Projects
Integrating timestamp to date conversion effectively can enhance the user experience on your website or application. Here are some effective methods to do so:
Case Study: E-commerce Applications
In e-commerce applications, displaying clear, human-readable dates for order timelines and delivery estimates is crucial. For example:
let orderTimestamp = 1633072800000; let orderDate = new Date(orderTimestamp); console.log("Order Date: " + orderDate.toLocaleString());Case Study: Scheduling Applications
In scheduling applications, you must ensure that users can understand their appointments easily. Converting timestamps to user-friendly formats is key. Here's an example:
let appointmentTimestamp = 1633169200000; // A future appointment let appointmentDate = new Date(appointmentTimestamp); console.log("Your Appointment is on: " + appointmentDate.toLocaleDateString() + " at " + appointmentDate.toLocaleTimeString());Conclusion
Converting a timestamp to a date in JavaScript is not just a technical necessity—it's a crucial skill that impacts the usability and clarity of your web applications. By understanding the methods discussed in this article and engaging with best practices, you can enhance your web design and software development projects.
Whether you're building an e-commerce platform or a scheduling tool, mastering these conversions will significantly improve user experience and functionality, ultimately leading to a more effective business operation.
timestamp to date javascript